ostream_iterator<T>
 |
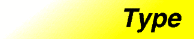 |
Category: iterators |
Component type: type |
Description
An ostream_iterator is an Output Iterator that performs formatted
output of objects of type T to a particular ostream.
Note that all of the
restrictions of an Output Iterator must be obeyed, including the
restrictions on the ordering of operator* and operator++
operations.
Example
Copy the elements of a vector to the standard output, one per line.
vector<int> V;
// ...
copy(V.begin(), V.end(), ostream_iterator<int>(cout, "\n"));
Definition
Defined in the standard header iterator, and in the nonstandard
backward-compatibility header iterator.h.
Template parameters
Parameter
|
Description
|
Default
|
T
|
The type of object that will be written to the ostream. The
set of value types of an ostream_iterator consists of a
single type, T.
|
|
Model of
Output Iterator.
Type requirements
T must be a type such that cout << T is a valid expression.
Public base classes
None.
Members
Member
|
Where defined
|
Description
|
ostream_iterator(ostream&)
|
ostream_iterator
|
See below.
|
ostream_iterator(ostream&, const char* s)
|
ostream_iterator
|
See below.
|
ostream_iterator(const ostream_iterator&)
|
Output Iterator
|
The copy constructor
|
ostream_iterator& operator=(const ostream_iterator&)
|
Output Iterator
|
The assignment operator
|
ostream_iterator& operator=(const T&)
|
Output Iterator
|
Used to implement the Output Iterator requirement
*i = t. [1]
|
ostream_iterator& operator*()
|
Output Iterator
|
Used to implement the Output Iterator requirement
*i = t. [1]
|
ostream_iterator& operator++()
|
Output Iterator
|
Preincrement
|
ostream_iterator& operator++(int)
|
Output Iterator
|
Postincrement
|
output_iterator_tag iterator_category(const ostream_iterator&)
|
iterator tags
|
Returns the iterator's category.
|
New members
These members are not defined
in the Output Iterator requirements,
but are specific to ostream_iterator.
Function
|
Description
|
ostream_iterator(ostream& s)
|
Creates an ostream_iterator such that assignment of t through it
is equivalent to s << t.
|
ostream_iterator(ostream& s, const char* delim)
|
Creates an ostream_iterator such that assignment of t through it
is equivalent to s << t << delim.
|
Notes
[1]
Note how assignment through an ostream_iterator is implemented.
In general, unary operator* must be defined so that it returns a
proxy object, where the proxy object defines operator= to perform
the output operation. In this case, for the sake of simplicity, the
proxy object is the ostream_iterator itself. That is, *i simply
returns i, and *i = t is equivalent to i = t. You should not,
however, rely on this behavior. It is an implementation detail,
and it is not guaranteed to remain the same in future versions.
See also
istream_iterator, Output Iterator, Input Iterator.
Copyright ©
1999 Silicon Graphics, Inc. All Rights Reserved.
TrademarkInformation